or C#’s Short-Circuit and Left-To-Right Evaluation
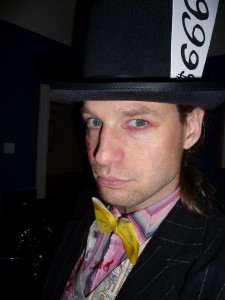
It’s all Rob Miles’ fault. Just before Friday lunch time he unleashed upon the world a work of unspeakable evil. There’s nothing wrong with the text of the article – Rob is his usual erudite self – rather that HDR-like picture of the Gulbenkian building. It nearly melted my face off.
It being Friday lunch time I thought I’d retaliate, not with more impossibly bright HDR photography but by doing unmentionable things to Anders Hejlsberg‘s wonderful language C#.
The challenge is this – the following is a reasonable enough piece of code but…
if (filmChoice == 1 && age >= 15) Console.WriteLine("Enjoy the film");
When you’re just learning to program it’s easy to make mistakes…
if (filmChoice == 1 && age >= 15) Console.WriteLine("Enjoy the film"); else Console.WriteLine("Access denied - you are too young");
There are 3 combinations of filmChoice
and age
that will lead you down the else path, not just when the customer is too young to see film 1.
So you need to break up the condition… or do you? Could you actually do it with just 1 if statement? Oh yes.
string film = "the other film", message = ", but you could see looper"; if ((filmChoice == 1 && (film = "Looper") != null && (message = "") == null) || age < 15 && ((message = "") != null) && filmChoice == 1) Console.WriteLine("Access denied to " + film); else Console.WriteLine("Enjoy " + film + message);
I'm actually practising my evil laugh right now. Later I will be uttering phrases like "No Mr Bond, I expect you to die!" and leaving the room allowing one of your dashing heroes to perform an improbable escape and ultimately foil my dastardly plan for world domination.
Seriously though, that code has been totally marinated in wrong sauce. Do anything like that in production code and your head will be on a spike in traitor's cloister faster than you can blink. Nevertheless it actually reveals some interesting and useful language features.
- C# always evaluates left-to-right
- C# always short-circuits evaluation
- Assignments have a result
- You can write some mind-bogglingly awful stuff in C# (not very useful)
Let's take a look at these one-by-one and by the end you should be able to work out how that particular atrocity manages to produce the right results.
Assignments Have a Result
The first thing we really need to understand is that assignment operations return a value. I know that appears to make no sense but it's true. The value they return is the value that's assigned. So variable = Math.PI
for instance assigns the value of PI to the variable and also returns the value of PI. Usually the runtime just throws this return value away.
Want to test this? It's easy enough to prove.
//let's be explicit about creating 2 doubles with values 0. double first = 0, second = 0; //assign the first to a constant first = Math.E; Console.WriteLine(String.Format("First [{0}], Second [{1}]", first, second)); //assign the second then assign the result of the assignment to first first = second = Math.PI; Console.WriteLine(String.Format("First [{0}], Second [{1}]", first, second)); //Output... //First [2.71828182845905], Second [0] //First [3.14159265358979], Second [3.14159265358979]
What's the practical use of this? Beyond being a handy syntax for assigning multiple variables the same values (or derivatives thereof) I'm not sure. Using the feature in conditional statements is convoluted, confusing and should definitely by avoided - even if the values are boolean.
if( bool1 = bool2 )
can easily be read as if( bool1 == bool2 )
but the two pieces of code do very different things. Conditional statements are not intended to modify things, they're intended to test them. Best to keep it that way.
Guaranteed Left-To-Right Evaluation
C# always looks at the left hand side of a conditional expression first, then it works its way towards the right. This might seem obvious but C did not guarantee this.
What use is it? Well it has a few uses on its own but they're mostly quite specialised. It's main use is when combined with the last language feature.
Short-Circuit Evaluation
Let's look at a simple if statement...
int a=5,b=7 if(a == 0 && b == 7) do_something();
We know it will be evaluated left-to-right so the term the runtime will look at first is a == 0
. We know that we set a
to 5
so this will evaluate to False
. The other term of the if statement, b == 7
is ANDed with the first - the entire expression can only evaluate to True
if both sides evaluate to True
.
The left hand side evaluates to False
so it doesn't matter what the right hand side evaluates to, it can't affect the outcome of the entire expression.
So the runtime doesn't bother with it. It can't affect the result so why bother wasting CPU cycles evaluating it?
The same is true of OR conditions.
int a=5,b=7 if(a == 5 || b == 7) do_something();
This time we've set a
to 5
so the first term of the expression evaluates to True
. This time though the second term is ORed with the first. True
OR anything is always True
so there's no point in the runtime evaluating the rest of the expression - so it doesn't.
What use is this? Well null checks are a really obvious one.
if(myObject != null && myObject.SomeProperty == someValue)
If myObject
is null
then the right-hand side will never execute. I must add a note of caution at this stage: some developers really don't like this construct. I think their main fear comes from C where the neither the order of evaluation nor short-circuiting were guaranteed by the language, so it could have evaluated this right-to-left or evaluated all the terms regardless, both of which would be pretty terminal.
There's also an argument that it's not clear. It is true that if badly written it can rather obfuscate the actual tests, but any badly written code can obfuscate the functionality (see the terrible if statement at the top of this article). My advice would be that if the relationship between the "gateway" test and the one that it's shielding is not immediately obvious then split it out into multiple if statements. Here's an example of something that's unclear :-
if(myFirstObject.SomeMethod() != 37 && mySecondObject.SomeProperty == someValue)
You might know that if SomeMethod()
doesn't return 37
then mySecondObject
will be null
but someone else reading your code probably won't and could easily break it. This should be more explicitly coded and properly commented.
Another use - and this one requires some thought - is optimisation. If you have a relatively expensive test and a relative cheap one, you might want to order your conditional statement carefully to save a few CPU cycles.
if(myObject.IsActive && Math.Sqrt(Math.Pow(targetX - myObject.X, 2) + Math.Pow(targetY - myObject.Y, 2)) < 100)
Square roots are quite expensive to calculate whereas the boolean is easy to test. So we put the boolean test in first and if this is False
then we won't bother evaluating the more expensive right hand side.
Conclusion
If you didn't know before you now have the tools to work out how my little abomination toward the top of the article works. It uses 3 language features.
- Guaranteed left-to-right evaluation means we can be sure what order an expression is going to be evaluated in.
- Short-circuit evaluation means that we know the runtime won't even run a test unless its outcome can affect the entire expression. We can use this fact to optimise code and even to stop the runtime executing tests that might throw an exception.
- The fact that assignments have a result isn't very useful and can cause a lot of problems. It's probably best avoided.
I resent the use of the phrase “HDR Like”. It was proper HDR. And as for nearly melting your face off, get one with a higher melting point….. I must admit the effect was turned up a bit (perhaps beyond 11) but I reckon that it was much more interesting than the original picture.
I do like your description though. I remember writing C code on the VAX, putting function calls and unary operators in expressions to try and get my head around the order of evaluation that the compiler dropped out. And I remember being very upset that I could write a C statement for which it was impossible to predict what result it would produce. I much prefer the way that C# works because statements are always predictable.
Sorry Rob – I couldn’t tell if it was proper HDR or a whizzo HDR-like effect. I’ve seen some pretty good post-processing effects lately. I’ve been known to mess about with HDR too.
I used to port a lot of C code; a lot from VAX to Solaris actually. The sheer amount of code that – being charitable to the developers – was tied to a specific compiler’s behaviour or their particular compilation settings was more than enough to convince me that everything in a language should be nailed down. It might block a few potential compiler optimisations but the price is worth it!